If you’ve looked into Machine Learning even a little bit, you’ve heard of Tensorflow, one of the most popular tools for designing, training, and using machine learning models.
https://www.tensorflow.org/
While you can certainly perform machine learning tasks without any pre-built tools, Tensorflow has become a beloved ecosystem of tools in the ML community to help people bootstrap their ML projects.
In this tutorial, I’ll show you how to get started using Tensorflow, and we’ll even build a pretty simple model to train and use.
If you want to see and run the finished product of what we’ll be making, you can check out this demo on Codesphere.
How Does Tensorflow Work?
The basic building blocks of Tensorflow, and ML in general, are tensors. Without getting too mathematical, tensors are groups of algebraic objects(Most commonly numbers) such as scalars, vectors, or matrices. Tensors can have any number of dimensions, making their formalization very helpful for traversing large and multidimensional datasets.
Tensorflow allows programmers to work with tensors in an incredibly easy and efficient way, and provides an additional library, called Keras, to build ML models out of tensors.
What We’ll Be Creating
To keep things simple, we’re going to make a neural network that can predict the outcome of an XOR(Either or) operator.
Our model is going to take in 2 values(Both booleans) and output 1 value (Also a boolean). We’ll be using 1 and 0’s in place of truth and false.
The inputs and outputs should follow the following logic table:
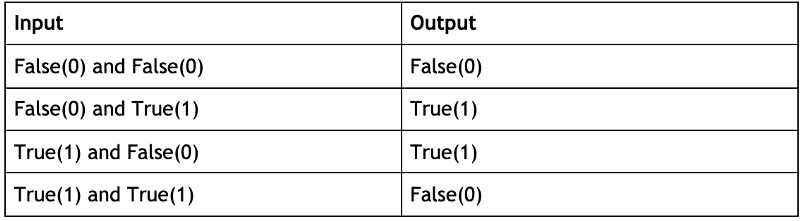
Could this be done much easier with some if statements? Of course. But the point here is to understand how to use Tensorflow and Keras so that you can end up creating something useful yourself.
Setting Up Our Environment
While this example should probably be fine on your local machine, building machine learning models can be incredibly memory intensive. It’s therefore helpful to use a virtual machine. Codesphere offers an online development environment so that you can code and deploy your apps seamlessly in the cloud.
The only necessary install here is Tensorflow, which we can install with:
pipenv install tensorflow
And we are only going to need one python file, which we’ll call main.py
Creating and Testing Our Model
Now let’s get coding!
Note the following steps:
- Define our training data
- Create our model
- Add layers
- Compile our model
- Fit our model to our training data
- Predict values
These are the steps you are going to want to take for virtually everything that you’re building in TensorFlow.
Also, note a few things which are going to differ based on the purpose and design of your model:
- Layer type: The type of layer you are creating(ie: Dense, Bidirectional)
- Activation function: A function that modifies the input of a neuron(ie: relu, sigmoid)
- Loss function: A function that measures the inaccuracy of a model in correctly predicting the training data(ie: mean squared error, categorical cross-entropy)
- Optimization function: The algorithm that is minimizing the loss value of the model. Is what is actually doing the training(ie: adam, stochastic gradient descent)
Next Steps
If all of these terms feel a bit more daunting than most of the programming that you’re doing, it’s because it probably is. ML is one of the areas of software engineering that requires more than some nicely worded stack overflow questions. It’s a lot of math, statistics, and CS theory.
That doesn’t mean, however, that you should just give up on learning it. There are plenty of books and online courses to help you learn!
When you’re ready to start building, you’re going to need a place to deploy your ground-breaking model. When that time comes, you can deploy in minutes to Codesphere, a zero-config cloud provider built to save you time.
Happy Coding!