Getting started with Web Sockets in NodeJS
Websockets don’t require a client request to fetch data from the server every time, fulfilling the need for real-time updates.
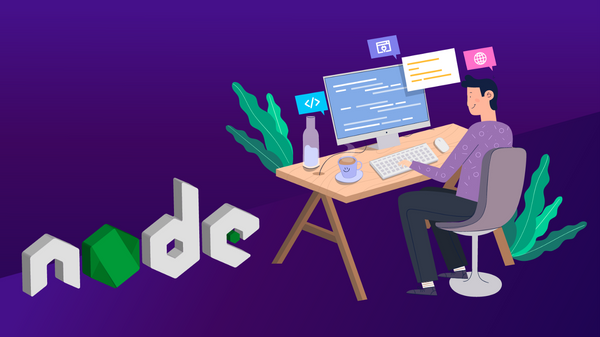
Table of Contents
In the day and age of live streaming, video conferencing, and remote work, one of the primary concerns of any user is latency. A seamless experience is the name of the game.
Traditional HTTP, being dependent on client requests, is simply not capable of fulfilling this need. They’re simply too slow. It needs to be upgraded, literally.
Let me introduce you to Websockets — An event-driven, web-friendly alternative to HTTP. Websockets don’t require a client request to fetch data from the server every time, fulfilling the need for real-time updates. Wondering how? Let’s find it out.
What are Websockets?
In simple terms, websockets are an upgrade to traditional HTTP.
Whenever a websocket request is made to regular HTTP, it is actually upgraded to a websocket connection. However, this happens only at the protocol level, meaning that your underlying TCP connection will remain the same as it was when working on HTTP protocol.
- In order to use a websocket, a client first sends a request to upgrade the server.
- If the server supports websockets, which these days it usually does, it will accept the request and switch the protocols from HTTP to websocket.
- Once the protocol switching is successful, the http server becomes a websocket server and a persistent connection is created between the client and the server.
- Next, the websocket server waits for an event to happen and once it does it performs the function attached to the event. For example, in a chat application you don’t have to place a request for the next message every time. Being a websocket connection (event-driven), the server simply pushes every new message (the event) it receives directly to the client.
Websockets are mostly used at places where real time updates are necessary. For example:
- Chat applications.
- Location based apps.
- Social feeds.
- Collaborative work.
- Multi player gaming.
- Live streaming.
- Financial and sports updates.
Each of the above renders an enhanced user experience thanks to WebSockets. Websockets always maintain their state (unlike HTTP, which is stateless) and utilize a full-duplex connection. Furthermore, headers in WebSockets are sent only once while sending the upgrade request. This is why WebSockets have speeds 5–7 times better than traditional HTTP.
Sounds exciting? It does but this is all well and good in theory. Nothing like the feeling than to see it in action and better yet implementing it yourself. So let’s get right to it.
Setting up the development environment
First things first, we need to set up our development environment by installing the required files and packages and creating our workspace. We will be using the ws library to create a simple chat application using websockets in NodeJS.
First, in our project folder create two folders named client and server.
Now fire up the node terminal and go to the server directory and run the following commands one by one
npm init -y
//initializes node and creates a package.json file
npm install websocket
//installs websocket library
npm install ws
//installs ws library — a websocket implementation
npm install express
//installs express for creating a simple http server
npm install nodemon
//installs nodemon package to track changes in our code and restart server
After this is done, create a file named index.js
inside your server folder. This index.js
will be our server-side javascript file.
Now we move on to the client folder. In the client folder create a file called index.html
and another file called script.js
. The HTML file will be the frontend for the application and script.js
will be the client-side javascript file.
Implementing the server
Now let’s code the server-side javascript. Add the following code to index.js
in the server folder.
Implementing the client
As we are done with the server-side, time to implement the client. We start with index.html
. Add the following code to it.
Moving to the script.js
file. Add the following code to it.
Websockets in Action
And there you have it!
When you deploy your app (make sure to run both the server and client sides), your HTML page will open up. If you type anything in the input box and click “Send Message” the server will echo it back to you. But where’s the fun in that? We want to see two clients chatting.
Open another browser or another instance of the same browser. Now go to the same URL as above.
Place both browsers side by side on your screen. Now when you send a message from one it will reflect in the other while echoing in the same as well. You can try the same with both browsers and it will work just the same. You can notice that the echo and the message in the other browser appear at practically the same time. That’s your WebSocket at work.
There you go! I hope you found this simple implementation of a chat application using WebSockets in NodeJS fun to work with. Get creative with it!
When you’re ready to show off your project, deploy it on Codesphere, the only cloud platform that takes the headache out of configuring your cloud services.
It only takes minutes to deploy!
That was it from our side. Let us know what amazing real-time application you’re going to build down below!