How to Start Using Typescript
One of the most significant innovations in web development in the past decade has been the shift towards Typescript. As more and more employers are looking to limit tech debt, learning Typescript is becoming essential to landing a job as a web developer. While dynamically-typed languages make life easier for the developer in the short run, it can become the reason for unwanted bugs that aren’t caught until runtime. We can avoid this problem with the help of TypeScript. Let’s take a closer look
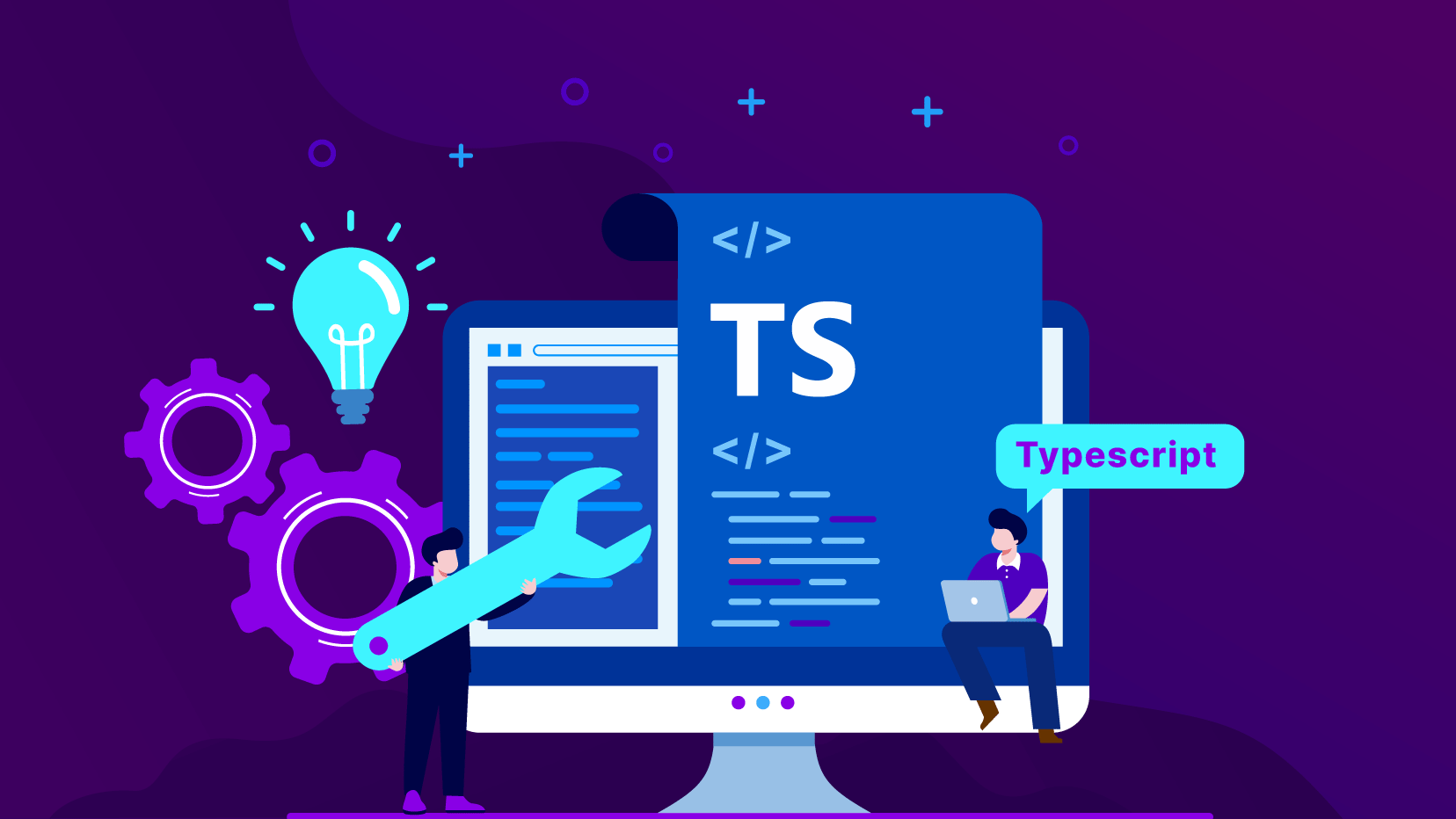
Table of Contents
One of the most significant innovations in web development in the past decade has been the shift towards Typescript. As more and more employers are looking to limit tech debt, learning Typescript is becoming essential to landing a job as a web developer.
While dynamically-typed languages make life easier for the developer in the short run, it can become the reason for unwanted bugs that aren’t caught until runtime. We can avoid this problem with the help of TypeScript. Let’s take a closer look
What is TypeScript?
In the simplest of terms, TypeScript is a superset of JavaScript. This implies that while conventional JavaScript code will work the same in TypeScript, it will also have an extra set of features making our code cleaner and better. TypeScript uses a compiler known as tsc to check for errors in the code and generates(technically known as emitting) the JavaScript equivalent for use in web applications.
TypeScript can also be referred to as a statically typed version of Javascript but we would be overlooking a plethora of other features that TypeScript has to offer. In the next section, we take a look at some of the advantages of using TypeScript.
Advantages of using TypeScript
TypeScript has the following features to offer:
- Strong typing - TypeScript places a lot of emphasis on the type of variables being used in the code. If the variable is assigned a value that doesn’t match the type, the TypeScript compiler tsc shows an error.
- Object-oriented features - TypeScript introduces a whole lot of object-oriented concepts that help make the code easier to manage.
- Compile-time errors - Since there is a compilation step involved, most of the errors get caught at compile time instead of run time.
- Emitting with errors - TypeScript will inform the developer about the potential errors in the code but it will make sure to generate the equivalent JavaScript to keep the development process running. The final decision is up to the developer.
- Great tooling - TypeScript provides access to a lot of great tools which help in editing, error checking, etc. as you type your code.
Let’s take a look at how we can do that with this simple demo that highlights a few of the above-mentioned features as well.
How to use TypeScript
Before moving on to the main demo and looking at a scenario similar to real-world applications let’s go through the basic building blocks of TypeScript first.
The Primitives
The usual JavaScript primitives number, string, and boolean are present here as well. In addition, you can do type annotation to fix the type of the variable at the time of declaration. TypeScript also infers the type when the variable is already defined. TypeScript also has a special type called any which is used to avoid type checking errors on a particular variable.
Functions & Objects
Functions also follow a similar pattern for type annotation where the parameter types are mentioned the same as for regular variables while the return type is mentioned between the parentheses and curly braces. Anonymous functions use a TypeScript feature called contextual typing where the type is inferred from the context of the function usage.
Objects are almost the same as JavaScript except for the fact that the types get inferred for every property inside it. Accessing properties that don’t exist gives a compiler error. TypeScript gives us the option of creating objects using type aliases (cannot be extended) or interface(can be extended).
Types on top of JavaScript
TypeScript adds some of its own types as well.
- Tuples are arrays that have their element types already annotated so we cannot save any other type on that location.
- Enum’s involve giving human-readable identifiers to numbers/strings. This makes it easier to manage code and avoid the hassle of memorizing fixed values.
- Unions allow you to use the same variable with multiple types of data without worrying about type errors. This is achieved by annotating multiple types on the same variable using the pipe(|) symbol.
Using Typescript in a Project
Since we are now familiar with the basic blocks of TypeScript, let’s build a small application and see how we can use it to write better code. This application will take two numbers as input and log their sum onto the browser console.
- Before we create the demo we need to set up TypeScript on our machine. Follow the instructions below to set up TypeScript on your machine.
https://www.typescriptlang.org/download
2. Create two files index.html and app.ts
. Make sure to call app.js
inside the HTML file. We will use the app.ts
to emit the app.js
file for use in the browser environment.
3. Create the index.html
as shown below. This file will contain two inputs for numbers and one add button. We will log the sum of the two numbers onto the console.
4. Inside the app.ts
file add the following code. This TypeScript code emits the JavaScript file which we will use to take the values from the DOM, adds them, and logs the result on the browser console.
5. To emit the app.js
, go to your terminal and run the following command to compile the app.ts
:
`tsc —target es2015 app.ts`
Make sure to run this inside the folder where your app.ts
is located. As mentioned earlier, tsc is the TypeScript compiler. This will compile our app.ts
and emit app.js
and generate error messages in case of any errors.
The target flag is required to make sure the JavaScript emitted follows the ES2015 standard. By default, tsc emits ES3 standard which is pretty old.
6. If you are using the code given above, it should work straight away. To see TypeScript in action, you need to remove the type annotations. Every time you make a change you need to compile the app.ts
again. Changes that don’t conform to TypeScript will generate errors. It will still, however, generate the JavaScript and you can even run it but it might give unexpected results.
We’ve just scratched the surface with what TypeScript has to offer! The biggest thing that you can do to get more familiar with typescript is begin to use it in your passion projects. The more comfortable you are working with Typescript, the easier time you’ll have using it to write clean code at your company.
Looking for a place to deploy that clean code? Check out Codesphere, the only cloud provider that makes deploying in the cloud as easy as testing locally.
Happy Coding!