Start Unit Testing Your Javascript with Jest
Most JavaScript developers either ignore or pay the least amount of attention to unit testing. Nevertheless, unit testing is crucial in weeding out issues before they drive away users. While we can always do manual testing, it is massively more efficient to perform testing by using testing frameworks. In today’s article, we’ll start unit testing using one such framework. -------------------------------------------------------------------------------- What is Jest? Jest is a popular JavaScr
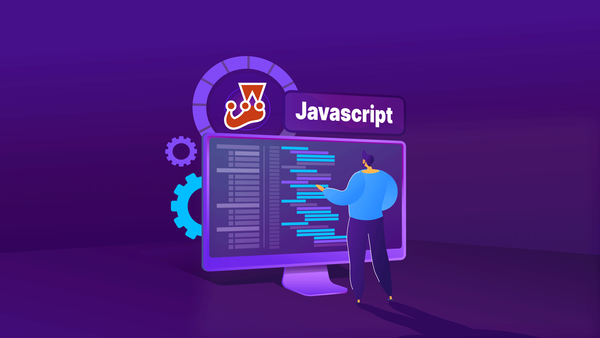
Table of Contents
Most JavaScript developers either ignore or pay the least amount of attention to unit testing. Nevertheless, unit testing is crucial in weeding out issues before they drive away users.
While we can always do manual testing, it is massively more efficient to perform testing by using testing frameworks. In today’s article, we’ll start unit testing using one such framework.
What is Jest?
Jest is a popular JavaScript unit testing framework that is great for minimizing the setup time for testing. It employs matchers to perform testing on the JavaScript codebase. Matchers take in an expected value and compare it with the output of an expect function which takes JavaScript code as input.
Jest then provides a detailed result of the tests to help make debugging easier by pointing to the exact place where it encountered the error in most cases.
Matchers
Some of the matchers that Jest includes are:
- toBe - For primitive data types to match by value.
- toBeLessThanOrEqual -Checks that the output value is below a certain value.
- toBeCloseTo - Primarily used to compare floating-point values. Since it doesn’t compare exact values it helps avoid false positives due to rounding errors.
- toMatch - Used for matching with regular expressions.
- toEqual - Just like the toBe matcher except that it is meant for reference data types such as objects.
- toContain - As the name suggests it checks if a particular element exists inside a given reference type such as an array.
Setting up Our Project
To set up our project, we’re going to create a new node project with:
npm init
We can then install Jest with:
npm install —save-dev
Next, in our package.json we are going to add the following script:
Finally, we’ll create two files: functions.js and functions.test.js
In general, for each javascript you want to test, you can just create an additional file called <Filename>.test.js
Using Jest in our Javascript Files
We can then implement Jest like so:
Running Jest
We can now run Jest with:
npm run test
And see the following output:
There you have it! We just set up our first test suite using Jest.
Once you’ve run your tests, fixed all your issues, and your code is ready to deploy, you can use Codesphere’s hassle-free cloud deployment. It takes just minutes to set up your project and have it live, so you can focus on the important task at hand: writing code.
Let us know what you’re building down below!