How to Build a GraphQL server with NodeJS and Express
Being able to work with APIs and write queries is crucial to any developer’s skill set. While working with REST APIs may be the standard, more and more teams opt to setup their API’s using GraphQL. In this article, we’ll set up our own GraphQL server with Node.JS(ES6) and write a few queries to gain insight into how GraphQL works. Let’s take a quick overview of the pros of using GraphQL before we begin to build our demo. -----------------------------------------------------------------------
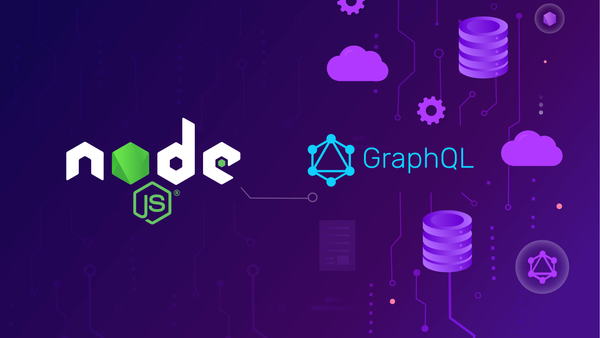
Being able to work with APIs and write queries is crucial to any developer’s skill set. While working with REST APIs may be the standard, more and more teams opt to setup their API’s using GraphQL.
In this article, we’ll set up our own GraphQL server with Node.JS(ES6) and write a few queries to gain insight into how GraphQL works. Let’s take a quick overview of the pros of using GraphQL before we begin to build our demo.
Advantages of using GraphQL
GraphQL is a query language used by developers to create flexible, and fast APIs which ensure that the clients receive only the data they requested. Other advantages of using GraphQL include:
- The ability to describe data offered by APIs on the server-side, as well as to send queries to the GraphQL server from the client-side.
- A declarative format with the query responses decided by the server which helps give better results.
- Queries that are composed of a hierarchical set of fields. It’s shaped just like the data that it returns making it intuitive to work with.
- GraphQL is a strongly typed query language. The type of data is defined at the server-side while describing data and validated inside a GraphQL type system.
Using GraphQL
We’ll be using GraphQL along with Express and NodeJS to create a simple server that returns data for an image or set of images depending on the query. To build a server with GraphQL we need the following:
- The GraphQL schema - which describes the type and hierarchy of a database. It also defines the query endpoints to which a client can send a request.
- The Resolver - to help attach a function to each query from the schema that executes when a client request contains that query.
- The Data - This will be the data that our server returns when it receives a query. For our demo purposes, we will create an array of image objects and return data as per the query.
Creating our Server
Without further ado, let’s build our GraphQL server using Express and NodeJS.
First, we’ll create our node project with
npm init
In our package.json
, we then need to add the following field:
Since we’ll be creating an ES6 Module.
Next, we’re going to install Graphql and Express with:
npm i graphql express express-graphql -–save
Finally, we’ll add the following javascript file to setup our server
Note that we used the buildSchema method provided by graphql to define our schema. The root method then is our resolver function. It attaches functions to the various queries available to the user. We need to define those functions in our file as well.
Using express we created an app variable that uses the graphql endpoint and the graphqlHTTP middleware. This middleware takes the resolver function and schema as parameters. We also set the graphiql option to true, which enables the GraphiQL in-browser tool which is used to test our endpoint by giving it queries.
Sending queries using GraphiQL
Inside our terminal, we can run the following command to start our server:
node graphql_server_demo.js
If you have named your file something else, obviously use that instead of the name above.
You can then go to http://localhost:5000/graphql to access GraphiQL, which will let us easily test queries
This should launch a GraphiQL interface that looks like this
In our demo code, we have two endpoints
- image: gets a single image by id
- images: gets images by a category
For the image endpoint, we can write a query like this
The $imageId is a variable that we can pass using the “Query Variables” section
The result for the above query will be
By removing or adding properties inside our query we can also modify what data is returned. Similarly, for our images endpoint, we can create a new query that will take the image category as a variable and return a list of images that match the category.
With the following parameter
Yields:
And there we have it! We’ve set up our own GraphQL server using Express and NodeJS. Of course, we’ve only scratched the surface of what GraphQL is capable of, and as the data we’re working with gets more complex, GraphQL is going to become more and more useful.
When you’re reading to deploy your server, check out Codesphere, the most intuitive cloud platform you’ve ever used.
Let us know what you’re going to build down below!